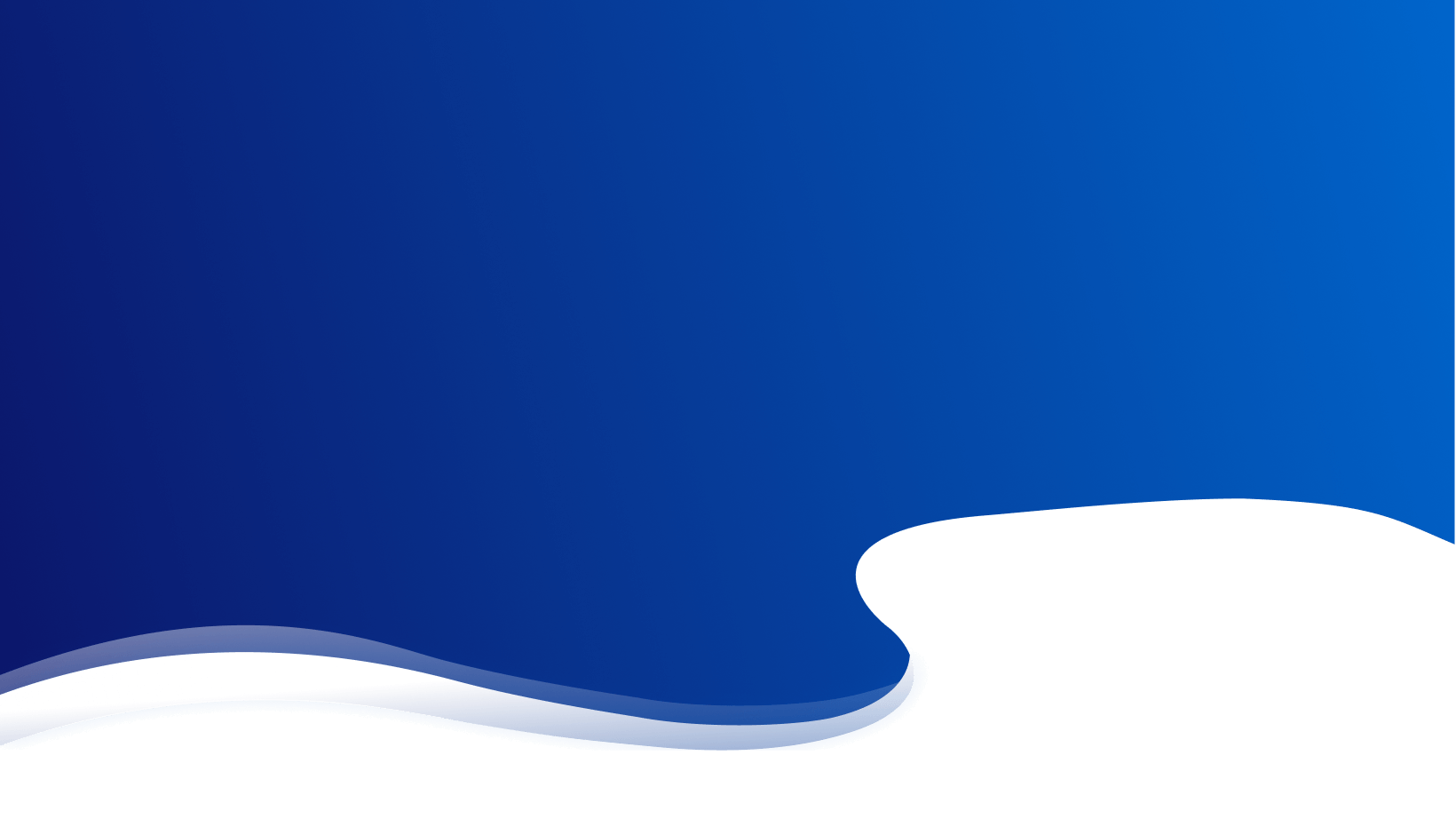
Salesforce Technical Architect Consultant
13 years developing Salesforce applications and over 30 years before that in various technologies.
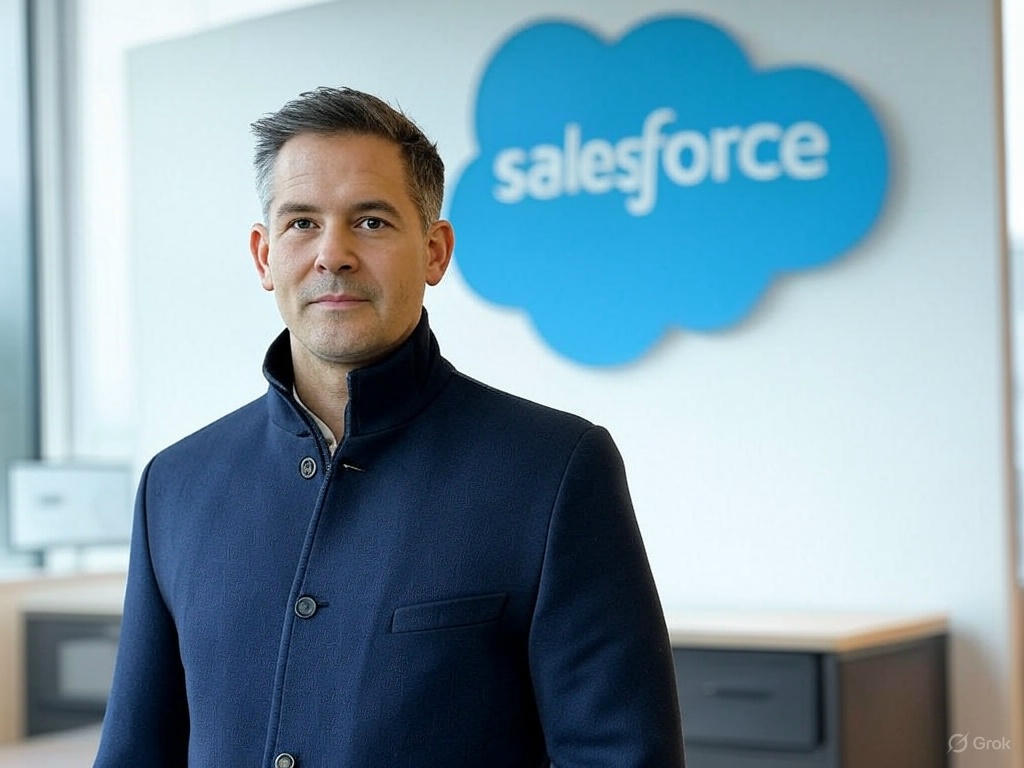

System Architecture
Architecture reivew, design and integation

Technical Leadership
Team leadership, code reviews and performance optimization

Advisory Services
System audits, technology stack and package selection

Strategic Planning
Roadmap development, governance framework and risk assessment

Specialized Services
Security review, data and scalability planning